THE COMPLETE IOS APP DEVELOPMENT WITH SWIFT
- Description
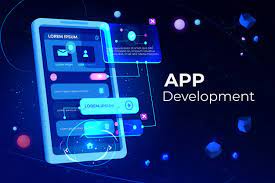
Course Objectives: In this program, you’ll not only learn how to build iOS apps, you’ll also learn best practices in mobile development, and gain mastery of Swift, an open-sourced object-oriented programming language. Through this course, you’ll gain the skills you need to become an iOS Developer.
*** 8 Sessions of 4 hours on Saturday/Sunday for 4 weeks *** Course run every month ***
Course Details:
Session 1: IOS/XCODE INTERFACE BUILDER
- Set up a new Xcode project.
- Use the Interface Builder to design and create the appearance of your app.
- Find your way around Xcode.
- Change the attributes of various UI elements.
- Arrange UI elements on screen by their setting their coordinates
- Size UI elements by changing their dimensions
Session 2: INTRODUCTION TO IOS APP DEVELOPMENT LANGUAGES
- What is Objective-C?
- What is SWIFT?
- Differences: Objective-C Vs. SWIFT
- SWIFT Programming Structure
Session 3: INTRODUCTION TO SWIFT PROGRAMMING
- Swift Playgrounds and become familiar with Swift syntax.
- Understand the data type system and how to use Strings, Integers, Doubles etc.
- Understand how to declare constants and variables using let or var.
- Swift functions and understand their input parameters and return
- Implement Class and Structure.
- Tuples, Optional, Enumerations, Operators, Statements, Arrays, Dictionaries (NS Dictionaries, NS Mutable Dictionary).
- IF-ELSE statements to control the flow of execution.
- Swift loops.
Session 4: APPLICATION DEVELOPMENT USING COMMON CONTROLS
- Creating IBoutlet, IBaction
- Using Text field, Text View, Button
- UISegmentedControl, Stepper, Switch, Slider, Progress bar
- UISwitchButton, UIStapper, UIActivityController
- UIView, UIStackView, UIActivityIndicatorView, UIAlertController (Alert and ActionSheet)
- Keyboard hiding, UIImageView, UIimagePickerController
- Text Sharing (UIActivityViewController)
Session 5: IMPLEMENTING BAR APPLICATIONS AND PICKERS
- Toolbar application
- Tabbed application
- UIPickerview
- UIImagePicker
- UIImagePicker for accessing iPhone gallery
Session 6: APPLICATION DEVELOPMENT USING ADVANCE CONTROLS
- Table view Application
- Custom Cell implementation
- UISearchController, UISearchBar
- UIGestures Controls
- Navigation bar, Navigationitem, bar button item
- Collectionview Application
- Webview Application
- Scroll Bar Application
- App icon, Splash Screen
- Validation [Text Field Validator]
- Higher order function
Session 7: IMPLEMENTING MULTIPLE SCREEN NAVIGATION
- App delegate, files Owner, Scene delegate
- Introducing multiscreen Storyboards
- UINavigationControllers
- Navigation using Segue
- Navigation using Storyboard ID PUSH | POP | Present | Dismiss
- Protocols and Delegation
Session 8: IOS DESIGN PATTERNS AND THE MODELVIEW-CONTROLLER (MVC)
- What is a design pattern and how is it used in programming.
- How to use the Model-View-Controller or MVC pattern for app development.
- Interface builder, XIB, NIB, Storyboard files
- UI and Code Debugging
Session 9: BUILDING RESPONSIVE USER INTERFACE | IOS AUTO LAYOUT AND SETTING CONSTRAINTS
- How to add constraints and understand how auto layout works.
- How to Pin and Align UI elements.
- How to create containers to configure advanced layouts. How to debug auto layout errors.
- Understanding what Xcode needs in order to correctly layout a design.
- How to use stack views to easily layout your UI.
Session 10: NETWORKING, APIS, DATABASE STORAGE AND THIRD-PARTY LIBRARY
- Directory Handling and UserDefaults
- Basic knowledge of Property List Files – Fetch and Load on table view
- JSON parsing – GET, POST
- Core data – CRUD
- All about Local Notification
- Singleton Class
- IPA (iOS App-store Package)
Session 11: IOS APPLICATION WITH THIRD PARTY INTEGRATION | CocoaPods
- What is a Third Party?
- What are Cocoa Pods?
- How to install / use Cocoapods?
- Working with third party controls using Cocoa Pod, IQKeyboardManager, Alamofire, and more.